Using the Invoke Method Activity in UiPath Studio
The Invoke Method activity enables you to call a method of a class and it can operate in two ways:
- using a Target Object, you can call an instance method on the object provided
- using a Target Type, you can call a static method of the provided type
When to use the Invoke Method activity?
There are a few situations in which you can use the Invoke Method activity:
- when you want to use a method which doesn’t return anything, so the call cannot be made from an Assign activity
- when the method you want to invoke has out parameters that need to be captured
Calling a static method
Let’s take the int.TryParse method as an example.
Start by defining 3 variables:
- input – of type string, this will be our string that needs to be parsed
- success – of type bool, this will indicate wether the parsing of the string was successful or not
- output – of type int (Int32), this will hold the parsed value
The first step in our test workflow will be to prompt the user for a string value and store that value in our input variable. For that purpose we use the Input Dialog activity.
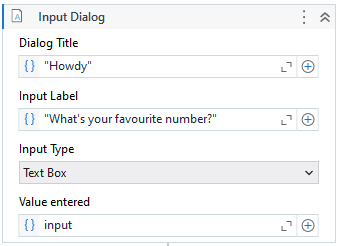
Next, we call the TryParse method using the Invoke Method activity. We configure the activity as follows:
- TargetType – here we select the class on which our static method is defined. We’re looking to parse an integer, so that will be System.Int32
- MethodName – we type in TryParse (without any quotes)
then in the Properties panel:
- Result – this is what the method returns. In our case, TryParse returns a boolean: true if the parsing was successful, false otherwise. Configure this to point to the success variable we have defined earlier
- Parameters – the TryParse method takes two parameters:
- the input, which is of type string, and In direction
- the output, which in an out parameter, so: Out direction, and type int
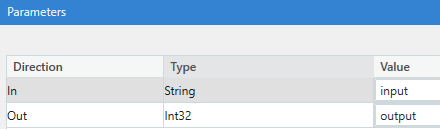
Now all that’s left to do is to use the success and output values. A simple example would be logging the parsed value in case of success and logging an error if the parsing failed (success equals false).
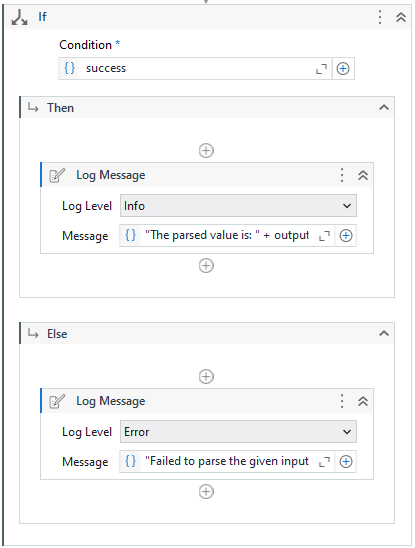
For a working example you can check out the InvokeStaticMethod workflow of the InvokeMethod example project.
Calling an Instance Method
Invoking an instance method is very similar to invoking static methods, the only difference being that instead of providing a TargetType, we need to provide a TargetObject representing the instance on which you want to call your method.
Let’s take a myList variable of type List<string> as an example. Set it’s default value to new List { “A”, “B”, “C” }.
Now let’s assume you want to Add a new item to that list. What you’ll need to do is to add an Invoke Method activity and configure it as follows:
- TargetObject – point this to your myList variable
- MethodName – type in Add (without any quotes)
- Parameters – configure an In parameter, of type string and value “D”
Let’s also reverse the list just for fun. Add another Invoke Method activity and configure it as follows:
- TargetObject – point this to your myList variable
- MethodName – type in Reverse (without any quotes)
Lastly, use a For Each activity to log the values inside myList.
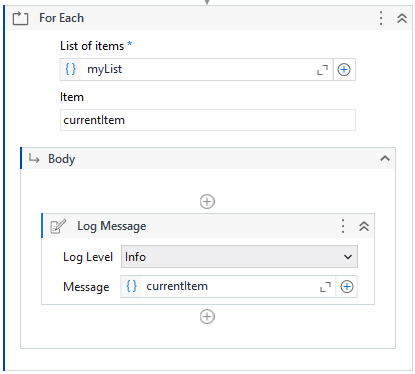
The output will be: D C B A.
For a working example you can check out the InvokeInstanceMethod workflow of the InvokeMethod example project.
Does Invoke Method work with private methods?
No. Invoke Method only supports calls to public methods. If you wish to make calls to private methods you can use the Invoke Code activity where you can use reflection to make the call.